Lecture Notes on Object-Oriented Programming
The Quality of Classes and OO Design
table of contents
Messaging is how work gets done in an OO system. Understanding messaging is a key part of being able to visualize how an OO program actually executes, and the relationship between the abstractions (objects) in an OO program.
A message has four parts:
- identity of the recipient object
- code to be executed by the recipient
- arguments for the code
- return value
The code to be executed by an object when it is sent a message is known variously as a method, or a function. Method is preferable.
Messaging an object may cause it to change state. The Elevator object will move when it is otherwise idle, and a user presses a floor button. But note carefully that what an object does when messaged depends on the state it was in when messaged. Thus the state of an object is a consequence of the history of messages it has received.
Objects messaging each other are what gets work done in an OO system. Rumbaugh says that two objects which can message each other have a "link" between them.
Remember, an object can't message another object without first having its name. So, how does an object find out the name of an object it wants to message?
- Name is global
- Name is part of the class of the messaging object
- Name is passed as a parameter to some operation
- Name is locally declared within object's method
- Name is provided as return value from message
How objects relate to each other depends on how they collaborate.
- Actor operates on other objects, but is never operated upon.
- Server is operated on by other objects, but never operates on other objects.
- Agent both and actor and a server.
A simple example to illustrate these various object roles is shown below
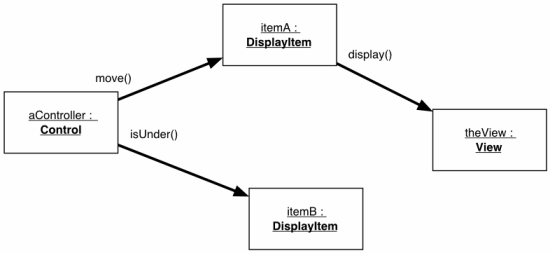
- aController represents an actor object, since it provides no service; is just asks other objects to do things.
- theView represents a server object, since it only takes message, doesn't generate them
- itemA represents represents an agent, since it provides a service to aController, and messages aView.