Lecture Notes on Object-Oriented Programming
The Quality of Classes and OO Design
table of contents
Class Relationship: Using
When a class definition uses another class as a parameter to a method, or declares an object of that class local to one of the member functions, then we say the first class is using the second.
Using is not the same as aggregation, since the class being used doesn't satisfy the whole/part question. The used class exists to help the first class.
For example, if a class method needed to keep track of a list of elements to do its works, then it could declare a List object as a local variable within the method. The List object will go away automatically when the method returns, just like any automatic (local) C variable. The class does not contain a list permanently, it simply has one method that uses a List.
Or another example: a class for calculating voltages, currents and power in an electrical circuit would need to deal with complex numbers a lot. A complex number class would be used to pass arguments to methods, hold temporary values, etc.
Class Relationship: Association
Classes that cooperate with each other, or are associated with each other through the model of our problem, need each others names to message back and forth. This is usually implemented in C++ by putting pointers to objects in class definitions, just like Graph had a pointer to NodeList. Association implies symmetry; both classes know each other and can message each other.
A Product class object may need to know the last sale that it was involved with. We could put a pointer to a Sale class object inside the private part of Product.
A Sale class object may need to know all of the Products involved with the Sale. We can put a pointer to a pointer (double pointer) in Sale that will let us store zero or more Products associated with a Sale.
Associations have a cardinality. They can be one-to-one, one-to-many, or many-to-many. Example: Each Product is part of 1 Sale; each Sale has many Products.
Associations are the most generic of the relationships between classes.
A class may also represent an association between two other classes. For example, a Skill and a Person are associated with one another. A Person can have some number of Skills, and a given Skill may be associated with some set of Persons.
To define the relationship, a Person-Skill class can have state such as proficiency and yearsExperience which tell how good a particular Person is at a given Skill, and how many years experience they have with it.
UML Representation
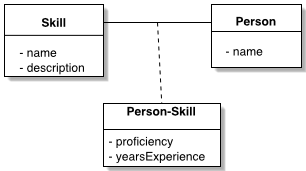